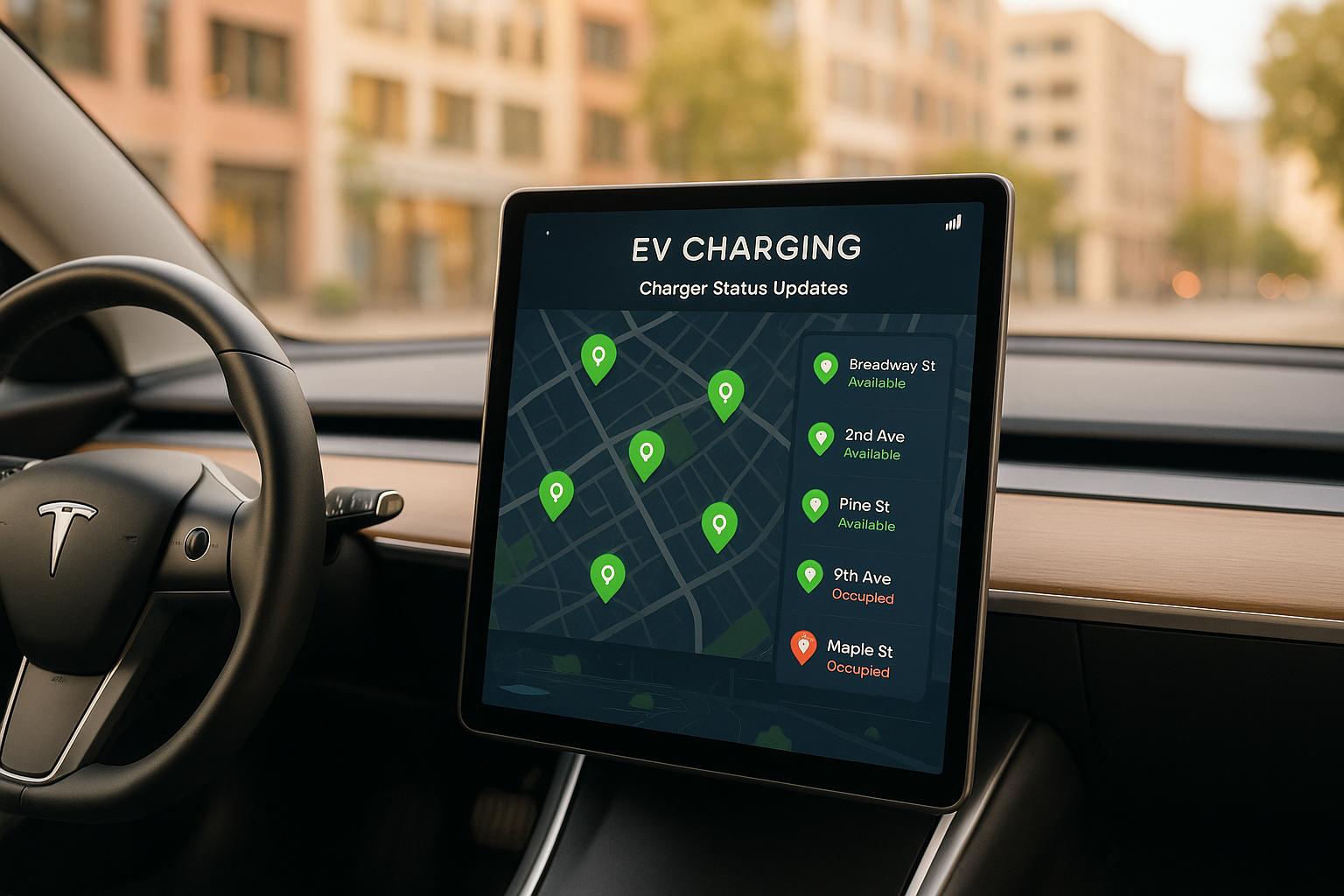
EV apps thrive on real-time charger updates. These updates help drivers avoid unusable chargers and long wait times, ensuring smoother trips. But achieving this requires solving technical challenges like connectivity issues and system reliability. Here’s how it works:
- APIs: Facilitate communication between chargers and apps, sharing data like battery levels and charging status.
- WebSockets: Enable instant updates with low latency, unlike traditional HTTP methods.
- Cloud Systems: Scale operations and ensure uptime using tools like AWS.
- Mobile Integration: Use WebSocket setups for Android (OkHttp) and iOS (Swift) to provide live updates.
- Offline Support: Cache data, queue actions, and auto-reconnect for seamless functionality.
- Security: Implement TLS encryption, OCPP standards, and regular penetration testing to protect user data.
Quick Comparison:
Technology | Purpose | Benefit |
---|---|---|
APIs | Data sharing | Real-time charger info |
WebSockets | Instant updates | Low latency, full-duplex comms |
Cloud Systems | Scalability and uptime | Efficient load management |
Offline Mode | Reliability in poor networks | Continuous usability |
Security Measures | Data protection | Prevent unauthorized access |
Real-time updates improve EV charging reliability but demand robust systems and strict security. Developers must balance innovation with protection to create dependable apps.
Is my car charging? How JUCR delivers real time EV charging status with federated subscriptions
Key Technologies for Live Status Updates
Delivering live status updates for charging stations hinges on a few critical technologies. Here’s a closer look at the key components that make it all work:
API Integration Methods
APIs act as the bridge between charging stations and user devices, enabling seamless communication. Through APIs, users can access real-time data such as battery levels, charging status, and temperature. They can also execute commands like starting or stopping a charging session. A well-designed API integration should efficiently handle these core data points:
- Battery levels and charging status
- Start/stop charging commands
- Temperature readings
- Load balancing data
WebSocket Setup for Live Updates
For real-time updates, WebSocket protocols outperform traditional HTTP connections. While HTTP is suitable for basic data requests, it lacks the persistent connection needed for instant updates. WebSockets, on the other hand, maintain an ongoing, two-way communication channel, enabling immediate data exchange between charging stations and user devices.
Feature | Traditional HTTP | WebSocket Protocol |
---|---|---|
Connection Type | Short-lived | Persistent |
Communication | One-way requests | Full-duplex |
Latency | Higher | Lower |
Real-time Updates | Requires polling | Instant push |
For instance, Slack uses WebSocket technology to deliver messages instantly. This same approach enhances EV charging systems by ensuring real-time data sharing, complementing API functionality.
Cloud Systems for Status Management
Cloud infrastructure plays a vital role in scaling and managing live status updates. Providers like AWS are leading the way, offering solutions that reduce IT costs and operational complexity. With the global number of public charging points increasing by 40% in 2023 compared to the previous year, scalable cloud systems are more important than ever.
AWS services, such as Fargate, allow Charging Point Operators (CPOs) to manage charging sessions efficiently without the need for physical infrastructure. Key features include:
- Containerized applications that adjust automatically to demand
- Load balancers to manage traffic distribution
- Edge computing for localized processing
- Redundant systems to ensure 24/7 uptime
Companies like Optiwatt and FlexCharging showcase how these technologies can be applied effectively. By leveraging EV APIs, they enable drivers and utilities to monitor battery levels and control charging sessions in real time, helping balance grid loads. These examples highlight the practical benefits of combining APIs, WebSockets, and cloud systems for live status updates.
Building Status Updates in Native Apps
When building native apps, creating real-time updates requires specific implementations that align with the backend technologies discussed earlier.
Mobile WebSocket Client Setup
To enable real-time updates, you’ll need to set up WebSocket connections for both Android and iOS apps.
For Android, you can use OkHttp for WebSocket support:
val client = OkHttpClient() val request = Request.Builder() .url("wss://your-websocket-server.com") .build() val webSocket = client.newWebSocket(request, object : WebSocketListener() { override fun onMessage(webSocket: WebSocket, text: String) { // Handle incoming charger status updates } })
For iOS, Swift provides a straightforward way to establish WebSocket connections:
let url = URL(string: "wss://your-websocket-server.com")! let session = URLSession(configuration: .default) let webSocketTask = session.webSocketTask(with: url) webSocketTask.resume()
These persistent WebSocket connections allow seamless real-time data exchange between the server and the app.
Status Display Updates
Once the WebSocket connection is set up, you can focus on updating the user interface with the incoming data. Use SwiftUI for iOS and Jetpack Compose for Android to create dynamic, responsive UI elements. Here are the key status elements to display and how often they should refresh:
Status Element | Update Frequency | Display Format |
---|---|---|
Charge Rate | Every 5 seconds | kW (1 decimal) |
Connection Status | Immediate | Connected/Disconnected |
Temperature | Every 30 seconds | °F (no decimal) |
Battery Level | Every 10 seconds | Percentage |
To ensure smooth updates, implement state management using view models. This approach allows the app to process WebSocket data streams efficiently, keeping the interface responsive without overloading the main thread.
Offline Mode and Error Handling
Network connectivity can be unpredictable, so it’s essential to build offline functionality that maintains usability. Here’s how you can handle offline scenarios:
- Cache the last known status: Store the most recent data locally to display when the connection is lost.
- Queue pending operations: For tasks like analytics or user actions, queue them and sync when the connection is restored.
- Reconnect automatically: Use exponential backoff to retry connections without overwhelming the server.
Here’s an example of a reconnection strategy in Android:
private fun reconnectWithBackoff(attempt: Int) { val delay = min(1000L * 2.pow(attempt), MAX_RECONNECT_DELAY) handler.postDelayed({ connect() }, delay) }
Different offline strategies can be applied based on the type of data or operation:
Strategy | Best For | Implementation |
---|---|---|
Online-only | Critical transactions | Direct server communication |
Queued | Analytics data | Background tasks with WorkManager |
Lazy | User preferences | Local storage with delayed sync |
sbb-itb-7af2948
Status Update Safety and Performance
Network Connection Stability
Ensuring stable network connections is crucial for EV charging apps, especially in tough environments like underground parking garages or remote rural areas. In these scenarios, cellular networks often outperform Wi‑Fi for maintaining reliable communication with EV chargers. Technologies like LTE‑M and NB‑IoT further enhance coverage in areas where connectivity can be a challenge.
Key features that support strong connections include:
Connection Feature | Implementation | Benefit |
---|---|---|
Message Persistence | Retain messages for 20 minutes; queue up to 100 messages | Prevents data loss during short outages |
Auto‑Retry Policy | 6 attempts with delays from 2 to 150 seconds | Maintains connection without overloading servers |
Connection Restoration | Automatic channel resubscription | Ensures smooth recovery after interruptions |
"PubNub’s automatic reconnection process is designed to handle brief network interruptions like driving through a tunnel, switching from Wi‑Fi to cellular, etc." – PubNub Docs
While maintaining stable connections is essential, securing these connections and the data they carry is equally important.
Data Security Measures
Once network stability is addressed, the next priority is safeguarding data. The Open Charge Point Protocol (OCPP) Security framework outlines three security profiles to meet varying needs:
Security Level | Features | Best Use Case |
---|---|---|
Profile 1 | Basic password protection | Suitable for development environments |
Profile 2 | TLS 1.2 or higher, server certificate | Ideal for standard deployments |
Profile 3 | TLS 1.2 or higher, client/server certificates | Best for high-security installations |
Key security practices include using TLS encryption, employing Public Key Infrastructure (PKI) to validate identities of vehicles and stations, and conducting regular penetration tests.
"Regular pentests on charging station products to identify vulnerabilities. These tests should cover hardware, software, and firmware components to ensure holistic security. Both physical access (attackers at the station) and network access should be assessed." – SEC Consult
By implementing these measures, operators can protect sensitive data and prevent unauthorized access, ensuring the reliability of their charging networks.
Meeting Industry Standards
Beyond securing connections and data, adhering to industry standards strengthens the performance and reliability of EV charging apps. Compliance with guidelines like VDE‑AR‑E 2802‑100‑1 ensures seamless Plug & Charge system functionality. For instance, a BMW X1e using an IoCharger IOCAH30 completed a TLS handshake in just 1.52 seconds.
To further enhance security, physical measures like tamper detection sensors and surveillance systems can protect charging stations from unauthorized access.
"OCPP Security implementation is critical for the security and stability of any charger network. Operators should verify that the chargers and management systems they choose implement these important security measures." – Wevo Energy
Conclusion: Building Better EV Apps
As we’ve explored, the success of EV charging apps hinges on two key elements: real-time data exchange and strong security measures. These apps must seamlessly blend technical advancements with a user-friendly experience to truly stand out.
Real-time updates are now transforming the EV landscape, enabling smarter charging decisions and better load management. By leveraging secure APIs and persistent WebSocket connections, as discussed earlier, developers can ensure smooth communication and dependable app performance.
"I think cybersecurity is extremely important and has not been discussed enough in the EV and charging industries. Everyone wants to talk about the next great feature and security is simply a far less ‘exciting’ topic."
The risks are real. In late 2021, a security breach in an EV charging app exposed sensitive data, including full names, addresses, and charging histories of over 140,000 users in the UK. This incident highlights why robust security measures aren’t optional – they’re essential.
To build apps that are both secure and scalable, developers must strike the right balance between innovation and protection. Adhering to industry standards, implementing strict security protocols, and maintaining stable network operations are non-negotiable steps for creating reliable solutions.
For those seeking expert support in crafting secure and efficient EV charging apps, Sidekick Interactive is here to help.
FAQs
How do WebSockets enhance real-time updates in EV charging apps compared to traditional HTTP methods?
WebSockets make real-time, two-way communication between an app and its server possible, delivering updates the moment they occur. This approach eliminates the need for traditional HTTP polling, where the app has to repeatedly request updates – a method that can lead to delays and put extra strain on the server.
For EV charging apps, WebSockets are a game-changer. They allow users to receive instant updates on charger availability, creating a faster and more dependable experience. In fast-paced scenarios like EV charging, where up-to-the-minute information is crucial, this technology ensures users stay informed and charging stations are used more efficiently.
How can developers ensure EV charging apps are secure and why does it matter?
To keep EV charging apps secure, developers need to focus on data encryption, such as using Transport Layer Security (TLS), to protect sensitive information like payment details and user credentials. Adding multi-factor authentication provides an extra layer of security, while securely storing data – preferably off the device – reduces risks. Developers should also rely on authorized APIs, safeguard the backend systems, and roll out regular security updates to close any potential loopholes.
These steps are critical in defending against threats like data breaches, unauthorized access, and financial fraud. A well-secured app not only protects users but also helps maintain trust and ensures the reliability of the entire EV charging network.
How can EV apps stay functional during network outages?
To keep things running smoothly during network hiccups, EV apps can offer an offline mode. This handy feature lets users start or continue charging sessions without needing an internet connection. For example, apps can pre-load crucial information like charger locations and user preferences, ensuring the app remains functional even when offline.
On top of that, chargers can be built to work independently, enabling charging to continue if it was already initiated or prepared before the network went down. By combining these features, EV apps can deliver a dependable and hassle-free experience, even in spots with unreliable network coverage.